Arithmetic Operators:
+ (addition), – (subtraction or negation), * (multiplication), / (division),
% (mod, (modulus or remainder)).
You can use the operators +, -, *, and / with both integral and floating-point data types.
You use % with only the integral data type to find the remainder in ordinary division.
When you use / with the integral data type, it gives the quotient in ordinary division.
That is, integral division truncates any fractional part; there is no rounding.
Since high school, you have been accustomed to working with arithmetic expressions
such as the following:
i. -5
ii. 8 – 7
iii. 3 + 4
iv. 2 + 3 *5
v. 5.6 + 6.2 *3
vi. x + 2 *5 + 6 / y
Arithmetic expression is constructed by using arithmetic operators and numbers. The numbers
appearing in the expression are called operands. The numbers that are used to evaluate
an operator are called the operands for that operator.
Unary operator: An operator that has only one operand.
Binary operator: An operator that has two operands.
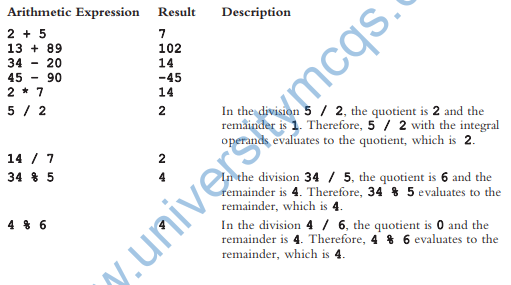
Practice Program:
The following C++ program evaluates the preceding expressions:
// This program illustrates how integral expressions are
// evaluated.
#include <iostream>
using namespace std;
int main()
{
cout << “2 + 5 = ” << 2 + 5 << endl;
cout << “13 + 89 = ” << 13 + 89 << endl;
cout << “34 – 20 = ” << 34 – 20 << endl;
cout << “45 – 90 = ” << 45 – 90 << endl;
cout << “2 * 7 = ” << 2 * 7 << endl;
cout << “5 / 2 = ” << 5 / 2 << endl;
cout << “14 / 7 = ” << 14 / 7 << endl;
cout << “34 % 5 = ” << 34 % 5 << endl;
cout << “4 % 6 = ” << 4 % 6 << endl;
return 0;
}
Sample Run:(Output)
2 + 5 = 7
13 + 89 = 102
34 – 20 = 14
45 – 90 = -45
2 * 7 = 14
5 / 2 = 2
14 / 7 = 2
34 % 5 = 4
4 % 6 = 4
// This program illustrates how floating-point expressions
// are evaluated.
#include <iostream>
using namespace std;
int main()
{
cout << “5.0 + 3.5 = ” << 5.0 + 3.5 << endl;
cout << “3.0 + 9.4 = ” << 3.0 + 9.4 << endl;
cout << “16.3 – 5.2 = ” << 16.3 – 5.2 << endl;
cout << “4.2 * 2.5 = ” << 4.2 * 2.5 << endl;
cout << “5.0 / 2.0 = ” << 5.0 / 2.0 << endl;
cout << “34.5 / 6.0 = ” << 34.5 / 6.0 << endl;
cout << “34.5 / 6.5 = ” << 34.5 / 6.5 << endl;
return 0;
}
Sample Run:
5.0 + 3.5 = 8.5
3.0 + 9.4 = 12.4
16.3 – 5.2 = 11.1
4.2 * 2.5 = 10.5
5.0 / 2.0 = 2.5
34.5 / 6.0 = 5.75
34.5 / 6.5 = 5.30769
Order Of Precedence:
When more than one arithmetic operator is used in an expression, C++ uses the operator
precedence rules to evaluate the expression. According to the order of precedence rules
for arithmetic operators,
*, /, %
are at a higher level of precedence than:
+, –
Note that the operators *, /, and % have the same level of precedence. Similarly, the
operators + and – have the same level of precedence.
For example, using the order of precedence rules,
3 * 7 – 6 + 2 * 5 / 4 + 6
means the following:
(((3 * 7) – 6) + ((2 * 5) / 4 )) + 6
= ((21 – 6) + (10 / 4)) + 6 (Evaluate *)
= ((21 – 6) + 2) + 6 (Evaluate /. Note that this is an integer division.)
= (15 + 2) + 6 (Evaluate –)
= 17 + 6 (Evaluate first +)
= 23 (Evaluate +)
Another Example:
In the expression:
3 + 4 * 5
*is evaluated before +. Therefore, the result of this expression is 23. On the other hand,
in the expression:
(3 + 4) * 5
+ is evaluated before * and the result of this expression is 35