Increment and Decrement:
These operators are used frequently by C++ programmers and are useful
programming tools.
Suppose count is an int variable. The statement:
count = count + 1;
increments the value of count by 1. To execute this assignment statement, the computer
first evaluates the expression on the right, which is count + 1. It then assigns this value to
the variable on the left, which is count.
To expedite the execution of such statements,
C++ provides the increment operator, ++, which increases the value of a variable by
1, and the decrement operator, ––, which decreases the value of a variable by 1.
Increment and decrement operators each have two forms, pre and post. The syntax of the
increment operator is:
Pre-increment: ++variable
Post-increment: variable++
The syntax of the decrement operator is:
Pre-decrement: ––variable
Post-decrement: variable––
Let’s look at some examples. The statement:
++count;
or:
count++;
increments the value of count by 1. Similarly, the statement:
––count;
or:
count––;
decrements the value of count by 1.
Suppose that x is an int variable. If ++x is used in an expression, first the value of x is
incremented by 1, and then the new value of x is used to evaluate the expression. On the
other hand, if x++ is used in an expression, first the current value of x is used in the
expression, and then the value of x is incremented by 1.
EXAMPLE:
The following example clarifies
the difference between the pre- and post-increment operators.
Suppose that x and y are int variables. Consider the following statements:
x = 5;
y = ++x;
The first statement assigns the value 5 to x. To evaluate the second statement, which uses
the pre-increment operator, first the value of x is incremented to 6, and then this value,
6, is assigned to y. After the second statement executes, both x and y have the value 6.
Now, consider the following statements:
x = 5;
y = x++;
As before, the first statement assigns 5 to x. In the second statement, the post-increment
operator is applied to x. To execute the second statement, first the value of x, which is 5,
is used to evaluate the expression, and then the value of x is incremented to 6. Finally, the
value of the expression, which is 5, is stored in y. After the second statement executes,
the value of x is 6, and the value of y is 5.
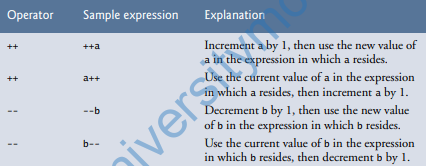