Selection statements are used to choose among alternative courses of action. For example,
suppose the passing grade on an exam is 60.
If student’s grade is greater than or equal to 60
Print “Passed”
determines whether the condition “student’s grade is greater than or equal to 60” is true
or false. If the condition is true, then “Passed” is printed, and the next pseudocode statement in order is “performed” (remember that pseudocode isn’t a real programming language). If the condition is false, the printing is ignored, and the next pseudocode statement
in order is performed.
The preceding pseudocode If statement may be written in C as
if ( grade >= 60 ) {
puts( “Passed” );
} // end IF statement
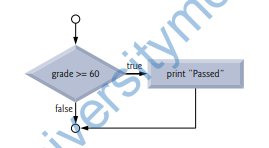
The expression is usually a logical expression. If the
value of the expression is true, the statement executes. If the value is false,
the statement does not execute and the computer goes on to the next statement in
the program. The statement following the expression is sometimes called the
action statement.
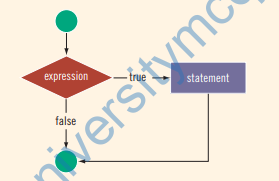
FOR EXAMPLE:
if (score >= 60)
grade = ‘P’;
In this code, if the expression (score >= 60) evaluates to true, the assignment statement,
grade = ‘P’;, executes. If the expression evaluates to false, the statements (if any)
following the if structure execute. For example, if the value of score is 65, the value
assigned to the variable grade is ‘P’.