While Loop:
An iteration statement (also called an repetition statement or loop) allows you to specify
that an action is to be repeated while some condition remains true. The pseudocode statement
While there are more items on my shopping list
Purchase next item and cross it off my list
The condition, “there are more
items on my shopping list” may be true or false. If it’s true, then the action, “Purchase next
item and cross it off my list” is performed. This action will be performed repeatedly while
the condition remains true.
The general form of the while statement is:
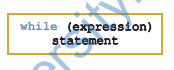
In C++, while is a reserved word. Of course, the statement can be either a simple
or compound statement. The expression acts as a decision maker and is usually a
logical expression. The statement is called the body of the loop. Note that the
parentheses around the expression are part of the syntax
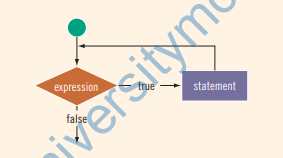
The expression provides an entry condition. If it initially evaluates to true, the
statement executes. The loop condition—the expression—is then reevaluated. If it again
evaluates to true, the statement executes again. The statement (body of the loop)
continues to execute until the expression is no longer true. A loop that continues to
execute endlessly is called an infinite loop. To avoid an infinite loop, make sure that the loop’s
body contains statement(s) that assure that the exit condition—the expression in the while
statement—will eventually be false.
EXAMPLES:
//1
i = 0;
while (i <= 20)
{
cout << i << ” “;
i = i + 5;
}
cout << endl;
Sample Run:
0 5 10 15 20
//2
As an example of a while statement, consider a program segment designed to find the
first power of 3 larger than 100. The integer variable product has been initialized to 3.
When the following code finishes executing, product will contain the desired answer:
product = 3;
while ( product <= 100 ) {
product = 3 * product;
}
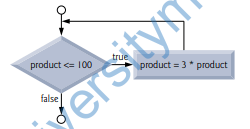