For Loop:
The while loop discussed in the previous section is general enough to implement
most forms of repetitions. The C++ for looping structure discussed here is a specialized
form of the while loop. Its primary purpose is to simplify the writing of counter-controlled
loops. For this reason, the for loop is typically called a counted or indexed for loop

The initial statement, loop condition, and update statement (called for
loop control statements) enclosed within the parentheses control the body (statement)
of the for statement. F
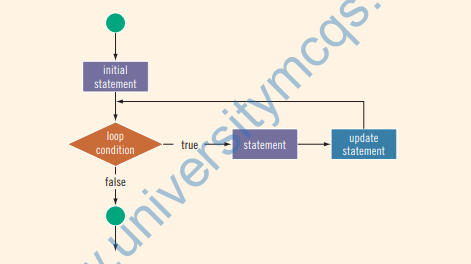
The for loop executes as follows:
1. The initial statement executes.
2. The loop condition is evaluated. If the loop condition evaluates
to true:
i. Execute the for loop statement.
ii. Execute the update statement (the third expression in the parentheses).
3. Repeat Step 2 until the loop condition evaluates to false.
The initial statement usually initializes a variable (called the for loop control, or
for indexed, variable).
In C++, for is a reserved word
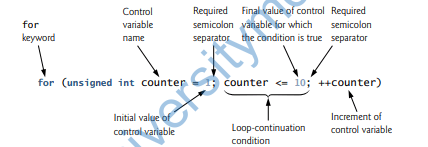
#include
int main(void)
{
// initialization, iteration condition, and increment
// are all included in the for statement header.
for (unsigned int counter = 1; counter <= 10; ++counter)
{
cout<<counter;
}
}
Another Example:
int main()
{
int sum = 0; // initialize sum
for ( int number = 2; number <= 100; number += 2) {
sum += number; // add number to sum
}
cout<<sum;
}
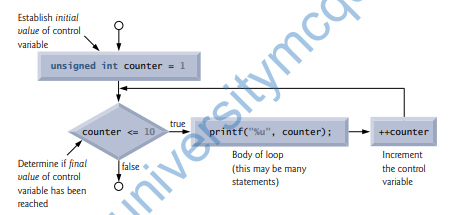