The expression is usually a logical expression. If the
value of the expression is true, the statement executes. If the value is false,
the statement does not execute and the computer goes on to the next statement in
the program. The statement following the expression is sometimes called the
action statement.
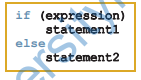
Take a moment to examine this syntax. It begins with the reserved word if, followed by a
logical expression contained within parentheses, followed by a statement, followed by the
reserved word else, followed by a second statement. Statements 1 and 2 are any valid
C++ statements. In a two-way selection, if the value of the expression is true,
statement1 executes. If the value of the expression is false, statement2 executes.
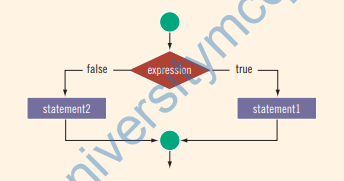
Let have an example:
If student’s grade is greater than or equal to 60
Print “Passed”
else
Print “Failed”
The uper statement in C++ is below:
if ( grade >= 60 ) {
puts( “Passed” );
} // end if
else {
puts( “Failed” );
} // end else
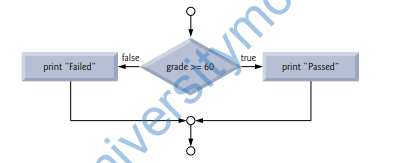
More Examples:
//1
if (hours > 40.0); //Line 1
wages = 40.0 * rate +
1.5 * rate * (hours – 40.0); //Line 2
else //Line 3
wages = hours * rate; //Line 4
//2
if (score >= 60)
cout << “Passing” << endl;
else
cout << “Failing” << endl;