Simple Data Types:
The simple data type is the fundamental data type in C++ because it becomes a building
block for the structured data type, which you start learning about in Chapter 9. There are
three categories of simple data:
1. Integral, which is a data type that deals with integers, or numbers
without a decimal part
2. Floating-point, which is a data type that deals with decimal numbers
3. Enumeration, which is a user-defined data type.
Integral data types are further classified into the following nine categories:
char, short, int, long, bool, unsigned char, unsigned short, unsigned int, and
unsigned long.
Difference Between char, short and int:
Why are there so many categories of the same data type? Every data type has a different set
of values associated with it. For example, the char data type is used to represent integers
between –128 and 127. The int data type is used to represent integers between
–2147483648 and 2147483647, and the data type short is used to represent integers
between –32768 and 32767.
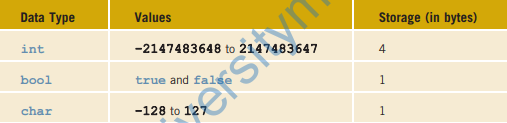
int DATA TYPE
This section describes the int data type. This discussion also applies to other integral data
types.
Integers in C++, as in mathematics, are numbers such as the following:
-6728, -67, 0, 78, 36782, +763
Note the following two rules from these examples:
1. Positive integers do not need a + sign in front of them.
2. No commas are used within an integer. Recall that in C++, commas
are used to separate items in a list. So 36,782 would be interpreted as
two integers: 36 and 782.
bool DATA TYPE
The data type bool has only two values: true and false. Also, true and false are called
the logical (Boolean) values. The central purpose of this data type is to manipulate logical
(Boolean) expressions. Logical (Boolean) expressions will be formally defined and discussed
In C++, bool, true, and false are reserved words.
char DATA TYPE
The data type char is the smallest integral data type. It is mainly used to represent
characters—that is, letters, digits, and special symbols. Thus, the char data type can
represent every key on your keyboard. When using the char data type, you enclose each
character represented within single quotation marks. Examples of values belonging to the
char data type include the following:
‘A’, ‘a’, ‘0’, ‘*’, ‘+’, ‘$’, ‘&’, ‘ ‘
Note that a blank space is a character and is written as ‘ ‘, with a space between the single
quotation marks.
Floating-Point Data Types:
To deal with decimal numbers, C++ provides the floating-point data type, which we
discuss in this section. To facilitate the discussion, let us review a concept from a high
school or college algebra course.
You may be familiar with scientific notation. For example:
43872918 = 4.3872918 * 107 {10 to the power of seven}
.0000265 = 2.65 * 10-5 {10 to the power of minus five}
47.9832 = 4.79832 * 101 {10 to the power of one}
To represent real numbers, C++ uses a form of scientific notation called floating-point
notation
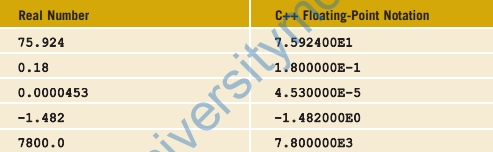
C++ provides three data types to manipulate decimal numbers: float, double, and
long double. As in the case of integral data types, the data types float, double, and
long double differ in the set of values.
Difference Between Float And Double:
float: The data type float is used in C++ to represent any real number between
-3.4E+38 and 3.4E+38. The memory allocated for a value of the float data type is
four bytes.
double: The data type double is used in C++ to represent any real number between
-1.7E+308 and 1.7E+308. The memory allocated for a value of the double data type is
eight bytes.
The maximum and minimum values of the data types float and double are system
dependent. To find these values on a particular system, you can check your compiler’s
documentation or, alternatively, you can run a program given in Appendix F (Header
File cfloat).
Other than the set of values, there is one more difference between the data types float
and double. The maximum number of significant digits—that is, the number of decimal
places—in float values is six or seven